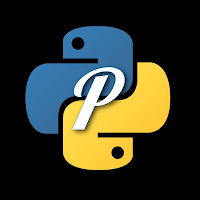
The base of the application is really simple; fetch the IP from http://checkip.dyndns.org/ and check if it's the same as the last time you've runned. If it has changed, send a message with pushover to your mobile phone.
I use crontab every 5 minutes to validate the IP.
Here's the script:
import re import os import uuid import json import socket import urllib, urllib2 import time def get_current_ip(): req = urllib2.Request('http://checkip.dyndns.org/') response = urllib2.urlopen(req) the_page = response.read() p = re.compile(r'(?P >ip<(?:[0-9]{1,3}\.){3}[0-9]{1,3})') m = p.search(the_page) return m.group('ip') # load settings current_ip = get_current_ip() uid = '' computer_name = '' last_ip = '' if(os.path.exists('/srv/crontab/oswos.settings')): with open('/srv/crontab/oswos.settings', 'r') as f: content = f.read() decoded = json.loads(content) uid = decoded.get('guid') computer_name = decoded.get('computer_name') last_ip = decoded.get('last_ip') else: uid = str(uuid.uuid1()) computer_name = socket.gethostname() data = { 'guid': uid, 'computer_name': computer_name, 'last_ip': current_ip } with open('/srv/crontab/oswos.settings', 'w') as f: json.dump(data, f) print 'uid : {0}'.format(uid) print 'computer_name: {0}'.format(computer_name) print 'last_ip : {0}'.format(last_ip) print 'current ip : {0}'.format(current_ip) if(current_ip != last_ip): API_URL = "https://api.pushover.net/1/messages.json" API_KEY = "api-key-here" curUrl = API_URL data = urllib.urlencode({ 'token': API_KEY, 'title': 'Oswos Notification', 'user': 'user-key-here', 'message': 'IP Changed from {0} to {1}'.format(last_ip,current_ip).encode('utf-8'), 'timestamp': int(time.time()) }) req = urllib2.Request(curUrl) handle = urllib2.urlopen(req, data) handle.close()
You see how easy it is to connect to Pushover? Building your own good working Android application is hard, so why not use the tools which are already there!
Just let me know if you have any tips / suggestions to extend this further.
Luuk